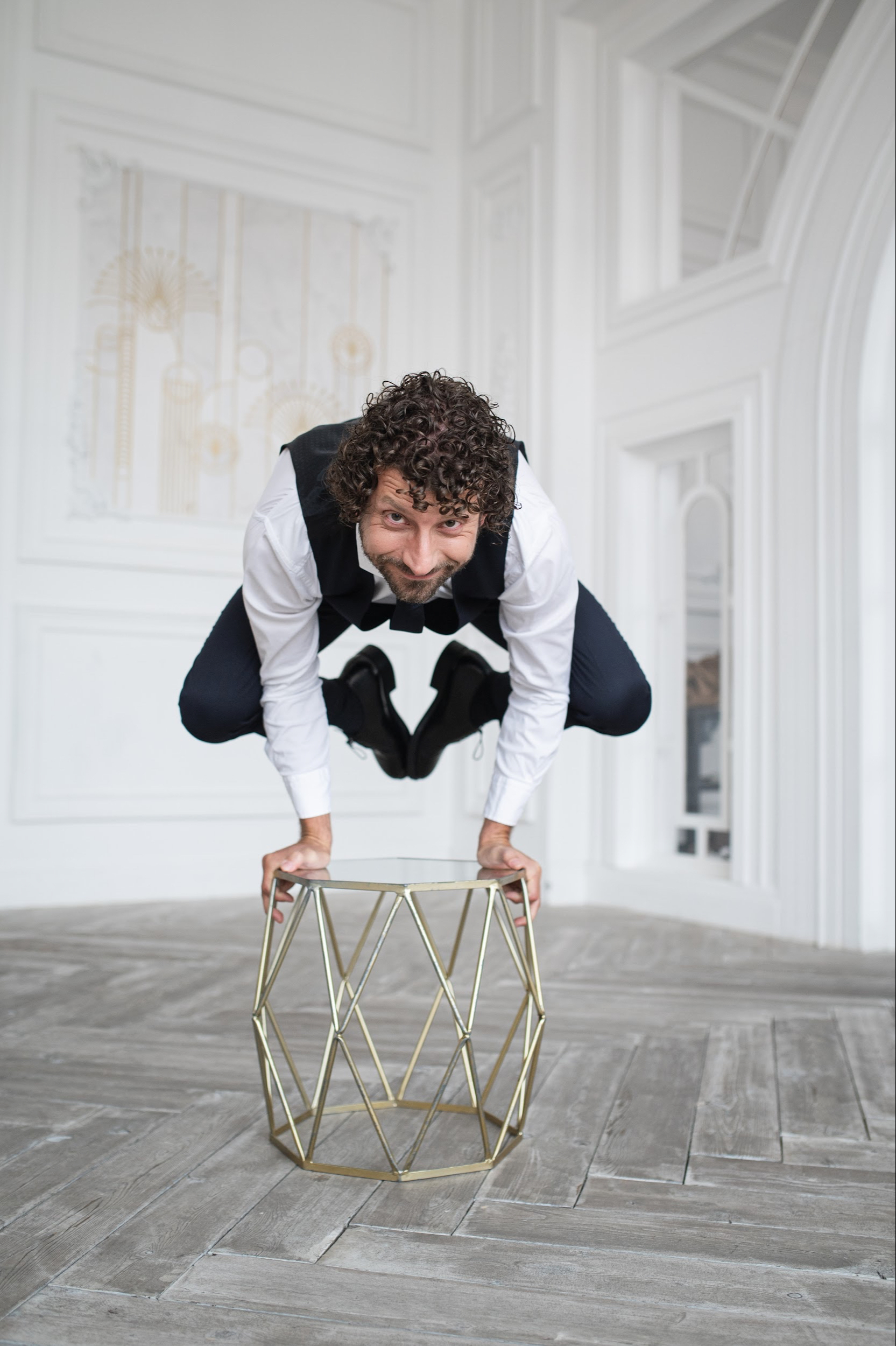
Learning Typescript
We all know Javascript, the language that transformed the web. Javascript has made it possible to move from websites to full-blown applications running in the browser, which has become the platform-agnostic “operating system” of our age.
Javascript has become so crucial to worldwide web development that a huge, whole ecosystem has evolved around it. Starting with the simplistic jQuery library for manipulating the DOM, the web ecosystem eventually grew to full-blown advanced frameworks such as React and Angular which have brought the state of the art in UI engineering to the world of web development. As Javascript grew in popularity, nodejs also made it possible to use Javascript in the backend as a server-side programming language.
In short, Javascript might have started as a language for writing tiny frontend scripts for the “web 2.0”, but nowadays it has earned its permanent place as one of the major programming languages next to the likes of Java and C#.
And yet, not all that shines is gold! Javascript is a dynamically typed programming language. This has a big disadvantage. When building large applications and reusing modules written by other developers, for example downloaded through yarn or npm, it is difficult to combine code together without making accidental mistakes. For example, passing an object with the wrong keys or the wrong values.
In the best of scenarios, we will need to work harder in order to cope with the need for extensive testing. Unit tests can be a solution, but for a huge application with a lot of code it will be a massive undertaking to write and maintain tests for the famed 100% code coverage (or close enough). And if the application changes all the time because of changing requirements, then we have twice the amount of work: change the functionality, and also change the tests, potentially for every single addition to the codebase.
But it gets worse (before it gets better)! Errors related to the wrong types in Javascript can have nasty bugs as a consequence. Sometimes a bug will not be easy to replicate, because the wrong types will hide for a while and only break something under some very specific circumstances that are hard to replicate.
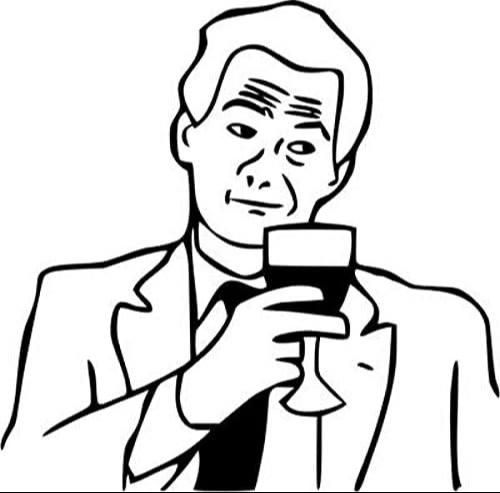
True story time: there once was a webshop that was very active during the weekend. Every Sunday evening the developers would get a bug report: orders could not be placed! The first responders would try and look into it, check the monitoring (backend was fine) try the usual quick fixes (restart the server) and then create a ticket for the regular team at the office to look into this. Monday morning first thing the critical issue would be checked, and it could not be replicated, so it was closed. After the third or fourth time, the devs started a proper in-depth investigation of the code, and discovered that the frontend data structure that contained the week day had a typo: “Sumday” instead of “Sunday”. Javascript, being a dynamically typed language, did not complain about it, the unit tests had 100% code coverage anyway but did not test all possible combinations of values, and yet this nasty bug remain undetected for way too long.
As Javascript applications grow bigger and bigger, this sort of issue becomes more and more likely. That’s where Typescript comes to the rescue. Typescript is all of Javascript, plus one little extra ingredient: an almost magical static type system.
A static type system is an AI that scans the whole source code, including dependencies, and makes sure that the right types are used in the right contexts.
A static type system will signal all errors such as:
a function is called with the wrong arguments
an object is missing a key
an invalid key is being used
a non-existent method or key is being used
a library is being used in the wrong way
and so on.
This AI actually uses a very advanced reasoning system, which is even capable of customization and flexibility. For example, in some cases, using an “Employee” object where a “Person” object is expected might be acceptable if we only stick to common attributes. In short, the “intelligence” part of AI is actually pretty solid!
And the best thing about this virtual assistant checking our code? It is always right, it is never tired, it never missed a night’ sleep, it is never distracted or stressed. In short, the type checker is always there for us and we can learn to lean on it as a trusted helper and advisor.
A little bit about types
There are different sorts of types. Some types carry some simple information. For example, the type checker will help us making sure that we do not use a string where a number is expected. Certainly useful, but not mind blowing.
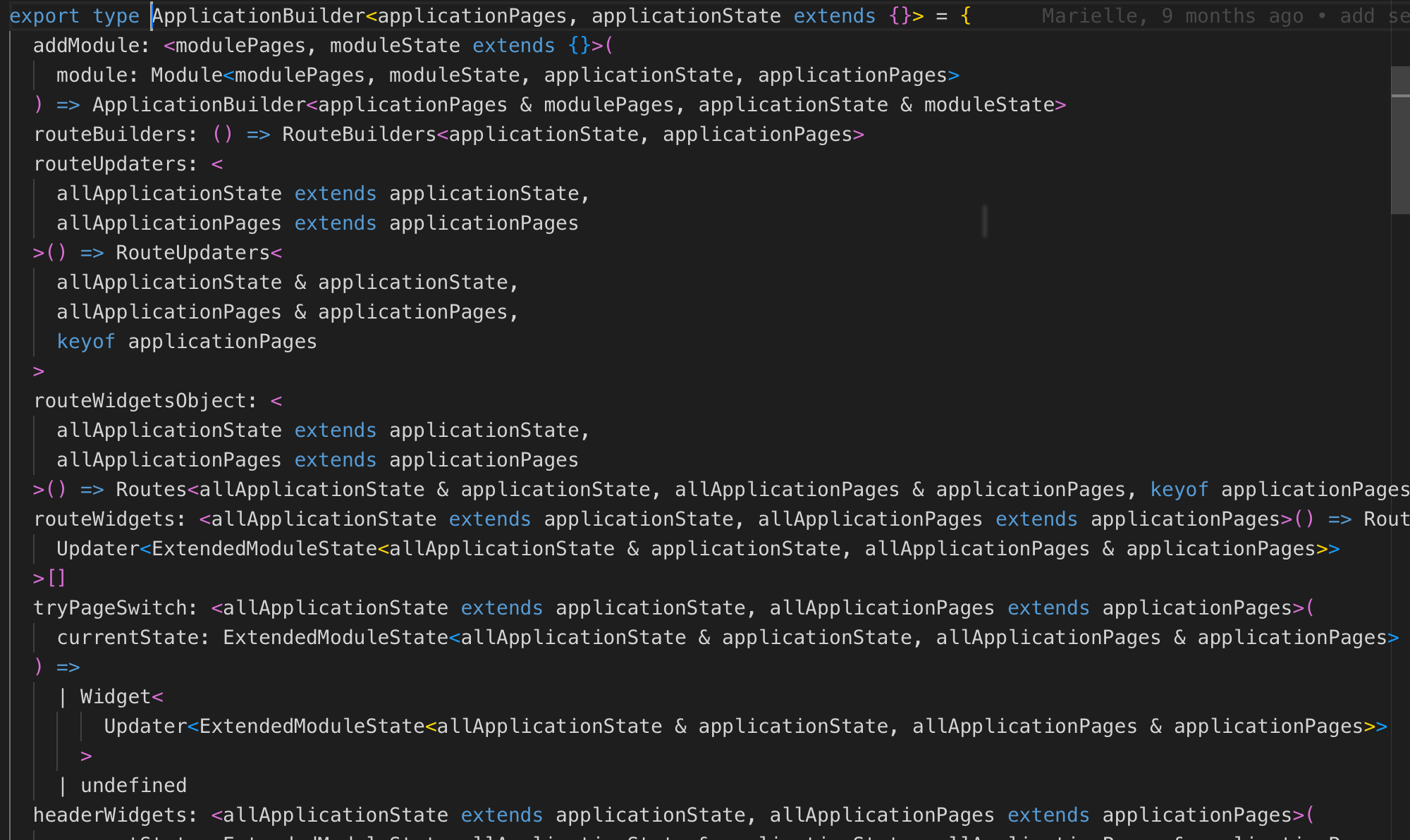
Advanced types are where the fun begins. Through advanced types we can get our virtual assistant to help with more and more complex topics so that the combination of different modules produces the combination of the right structures. For example, we could say that if we include a login module and a shopping module to our application, the state of the application should automatically include the login data and the shopping cart data, so that our virtual assistant can keep track of this very useful high level information about the whole application state for us.
And let me tell you: the number of bugs this thing can learn to catch is out of the world!
In order to help my colleagues at Hoppinger, and also to share this with the world, I created an Introduction to Typescript course. The course is meant for people at all levels. Beginners will be able to learn the language basics, and intermediate and advanced practitioners will find plenty of inspiration to strengthen their understanding of the language and its patterns. We will talk about:
variables, conditional statements, basic loops, and primitive types
functions, lambdas, and recursion
custom types
generics and standard generic libraries (Array, Promise, Immutablejs)
classes and object orientation
basic object oriented design patterns for practical use
Each topic features full length videos with a theoretical explanation (hands on with live coding, always), as well as many exercises solved for you in real time.
After completing this course, you will be able to access more courses from the academy. Soon we will publish courses on React, Docker, SQL and NoSQL databases, Strapi, and many more interesting topics.
Happy learning!